Current status
Functioning
- Main menu
- Godot's project upgrade tool
- Signal / Connection adjustments
- Commenting out theme and font code
- Number fall animation works on Linux and Windows
- `get_viewport().get_size_override()` -> `get_viewport().size`
- OS.get_name() output changed
- Basic game loop
- Start new game
- User input
- Error checking and tracking
- Game time tracking
- Game finished
Non-Functioning / Unknown
- DB Browser
- Font selection
- Generator
- Number fall animation - animation preload
- Options menu
- Save / Load game
- Theme modification
Next spoonful of elephant ice cream
The basic game loop works, but everything looks terri-bad. Next bit of ice cream is getting back into the Options menu and get the appearance customization working again.
With a bit more theme-related code commented out, the Options menu is loading.
Drop down menus are blank, theme and font changes are ineffectual (due to commented code and 4.0 changes to both). But number spinners are still working, as are the ConfigFile CRUD operations, so updating the verbosity level a bit will give me a better sense of what's going on with in the system.
Message queue
Presently, messages run through functions attached to a "Global" Singleton which pushes them into a message Array. A separate timer prints and removes the message from the front of the array. There is a keyboard shortcut (F12) to clear the queue by skipping the timer and cycling over the array.
Is this the most efficient way to do this? Probably not. As the queue fills up the remove_at function becomes less and less efficient. Figure it's better to have this inefficiency at the read, since the write operations may hold up the game loop, plus the read is consistently delayed with the timer anyway.
But why?
[output overflow, print less text!]
The console built into the Godot editor will truncate messages sent by the application if there's too much coming in at once. The message queue and timer should prevent the system from truncating messages.
It's also possible to load the editor from the command line in your project with `godot -e` (so long as godot's binary is in your path, otherwise you have to call it with an absolute path, but don't forget the `-e` to invoke the editor).
Messages get replicated to the command line without being truncated when godot is invoked this way.
Review of options
- General
- Difficulty
- Number spinner: Number can change within expected range, message output marks every number selected as "out of range".
- Drop-down: Empty
- Input Orientation: Empty
- Computer controlled actions
- Check for errors automatically: Working as expected
- Compute notes: Working as expected
- Clear conflicting notes: Working as expected
- Show unique check warning: Check pending difficulty fix
- Dev / Debug
- Enable console: Console fails at `Global.console.insert_text_at_cursor`
- Invalid call. Nonexistent function 'insert_text_at_cursor' in base TextEdit.
- Verbosity
- Number spinner: Working as expected
- Drop-down: Empty
- Enable console: Console fails at `Global.console.insert_text_at_cursor`
- Difficulty
- Appearance
- Theme: A lot has been commented out to get to this point.
- Left arrow: Works as expected
- Selection: Initialization is commented out, but changes work as expected
- Right arrow: Works as expected, alignment if off.
- Remove button: Pending dialog box fixes
- Export button: Pending dialog box fixes
- Import button: Pending dialog box fixes
- Font: A lot has been commented out to get to this point.
- Color: Works as expected
- Left arrow: Works as expected
- Selection: Initialization is commented out, but changes work as expected
- Right arrow: Works as expected, alignment if off.
- Size:
- Left arrow: Works as expected
- Slider: Works as expected
- Right arrow: Works as expected, alignment if off.
- Colors: Changes may work, but saving involves modifying the theme
- Border: Missing
- Playfield: Works as expected
- Table: Missing
- Cell: Works as expected
- Background dropdown: Empty
- Base: Works as expected
- Flash Correct: Changes take effect but colors don't flash correctly
- Flash Incorrect: Changes take effect but colors don't flash correctly
- Highlight: Works as expected
- Locked: Works as expected
- Selected: Works as expected
- Solved: Works as expected
- Theme: A lot has been commented out to get to this point.
- Audio
- Music
- Left arrow: Works as expected
- Selection: Work as expected
- Right arrow: Works as expected, alignment if off.
- Levels
- Music Slider: Slider works and DB scale seems appropriately exponential (based on messages), but Music is either on or off.
- Sound Effects: Slider works and DB scale seems appropriately exponential (based on messages), but sound effects is either on or off.
- Mute
- Music: Works as expected
- Sound effects: Works as expected
- Music
- Video: No controls yet.
- About
- Generator Button: Pending Generator scene fixes
String vs str()
It seems the number of constructors associated with String, the class, has decreased. Instead, the Global GDScript method str() is designed for taking variables and converting them to a String.
Animations
Input buttons have animations that control the view, specifically a pulse animation that adjusts the button's modulation based on user configuration. This is one of the errors that's causing issues, especially on a machine that doesn't have a pre-existing config and theme built from the 3.5 branch.
Previously, Animations where attached to the AnimationPlayer in the scene or object. Sudoku's old code would copy the animation from the player, adjust the keyframe color, remove and re-add the animation to the player.
With 4.0 this no longer works. I think it was the project upgrade tool that renamed previous calls to the AnimationPlayer's `remove_animation()` to `remove_animation_library()`.
AnimationLibrary
There seems to be very little existing documentation on the new AnimationLibrary. It seems to be a new abstraction layer for animations so they can be shared (and adjusted) between nodes.
Sudoku's Main Menu currently has 7 input buttons in the editor (fewer are shown in the built version). Prior to Godot 4.0 each of the 7 input buttons would have a separate "pulse" animation. With the new AnimationLibrary, animations are built in shareable libraries that the 7 input buttons all reference.
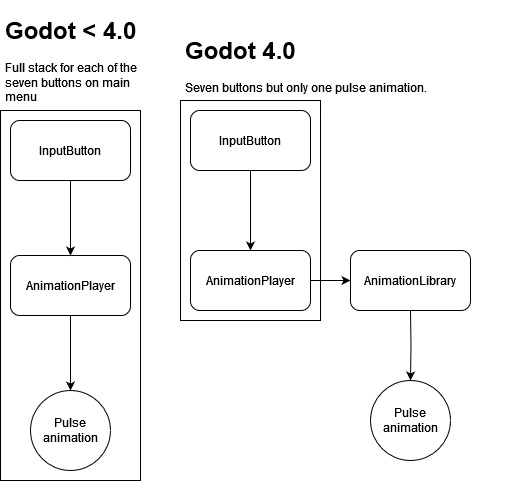
There might be a better way to manage it, but for now the InputButton will check the relevant keyframe to see if a change is needed before pushing an update. Which will reduce repetition if nothing else.
How to create a AnimationLibrary
Select the Animations tab near Output and Debugger, it may be necessary to select an AnimationPlayer first. Next to the play forward and backward buttons is another button marked "Animation" which shows a drop-down menu, click "Manage Animations..." to bring up the Edit Animation Libraries dialog. In here a new library can be created and named along with the expected animations, say "Pulse" and "Reset". Finish off by clicking the OK button to return to the main editor.
Select the animation to edit from the drop-down next to the Animation Button, say "Effects/Pulse". Add a property track, and add the key frames as desired. For the Pulse animation the Modulation property gets modified. Set the first keyframe at 0 seconds, the second at 1 second, the final at 2 seconds, and set the Animation Looping style to loop.
In the code, the animation gets updated based on the theme color, while the first and last keyframe stay the same as the reset animation: White with full alpha.
Version
Godot: 4.0.3
Reference
- Godot's Animation Tutorial
- Godot's Retargeting 3d Skeletons
Comments